First Program in Python
As any programming language has common components like variables, control structure, loops (iterations), Arrays, Functions, Classes, Constructor etc. During this article we will learn syntax for the same in Python.
Variables :
- In python variable names are case sensitive like C,C++, Java,C# etc. so that X is not x here.
- Variable name can contain any character, digit and _ but can not start with number.
- No space is allowed between variable name.
- Variables in python is dynamically typed like PHP so no data type is required at variable declaration.
- exaples are roll_no=112, name=”XYZ”, percentage=98.33 etc.
- Mostly Entire Capital word is used to declare Constant variables PI=3.1416
Comments :
- Character # is used for comment in python
- Python does not have multiline comment.
- ex. x=20 # value of x
Data Types
Integer :
When you assign any integer value to any variable then it becomes integer variable. In python there is no limit for Integer value it is depends on System memory.
x=101100101113343434343
y=3423423424234242423424
z=x+y
print(z)
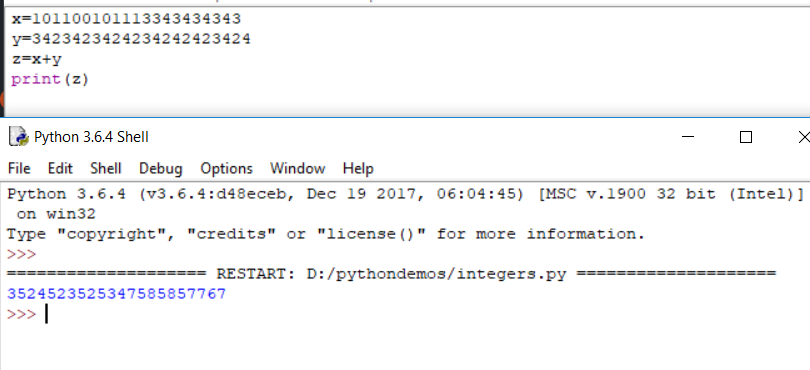
Some other types are :
x = 903 # integer
pi = 3.14 # double float
msg = "hello" # string
lst = [0,1,2] # list
tpl = (0,1,2) # tuple
#fl = open(‘hello.py’, ‘r’) # file
print(x,y,pi,msg,lst,tpl)
903 344444 3.14 hello [0, 1, 2] (0, 1, 2)