Dictionary in Python
As if you are aware of PHP Associative array, then you can easily compare concept of dictionary in python . Dictionary is a collection of values with their key.
Dictionaries are unordered sets in Python. Dictionaries don’t support the sequence operation of the sequence data types like strings, tuples and lists
marks ={'Sachin':98,'Virat':35,'Rahul':55}
print(marks)
print(marks['Sachin'])
for mark in marks.values():
print(mark)
for key,val in marks.items():
print(key,' got ',val)
marks['Rohit']=94
print(marks)
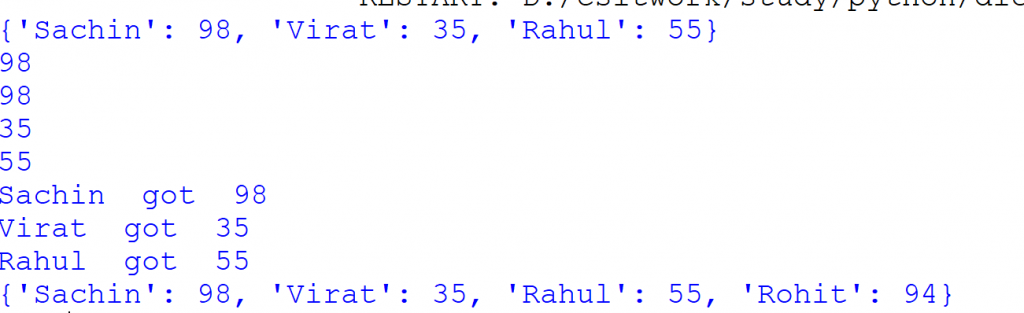
Points to remember : Key is Case Sensitive Sachin is not same as sachin here. It will raise an error when you try to retrieve the values with wrong key.
In case of assignment, it will update value of existing key or append the new key (if not exists)
You can remove any pair by using pop()
eg.
m= marks.pop('Virat') # Here M will be 35, Virat will be removed
print(m)
print(marks)
Another method which can delete a pair is del
del marks['Rahul'] #will delete Rahul pair
print(marks)
In both method it will raise an error if key is not founded. So it is better practice to check if the key is exists or not.
#To check for key
key='Jadeja'
if key in marks:
print('Founded')
else:
print('Not Founded')
To Clear All Elements from Dictionary
marks.clear()
print(marks)
Final Program and Output for Dictionary Tutorial
marks ={'Sachin':98,'Virat':35,'Rahul':55,'Jadeja':34}
print(marks)
print(marks['Sachin'])
for mark in marks.values():
print(mark)
for key,val in marks.items():
print(key,' got ',val)
marks['Rohit']=94
print(marks)
m= marks.pop('Virat') # Here M will be 35, Virat will be removed
print(m)
print(marks)
del marks['Rahul'] #will delete Rahul pair
print(marks)
#del marks['Rahul'] #will Generate Error as No Key Now named Rahul
#To check for key
key='Jadeja'
if key in marks:
print('Founded')
else:
print('Not Founded')
marks.clear()
print(marks)
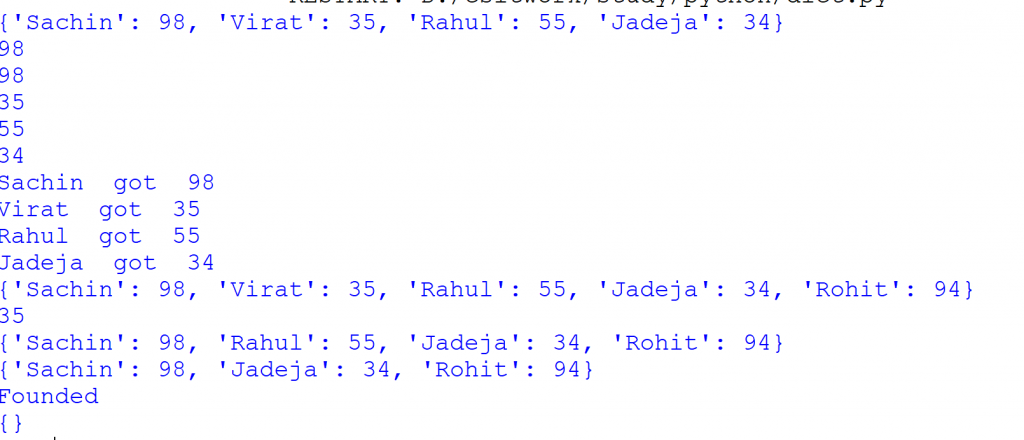