Variables and Constant in PHP
In any Programming language variables are used to store data for temporary period or scope. In php variables are named with $ sign. In PHP Auto Declaration of variable is done when you assign a value to variable. No need to specify data type like c,c++ or java.
ex. $x=20; $nm=”OCJP”; etc.
Based on location of the variable and prefix attached with variable, Scope of the variable is defined.
<?php
$x=11;
echo "<br>X is ".$x; //. is for concatenate
function showData()
{
$y=21;
echo "<br/>Value of Y in Function ".$y;
}
echo "<br/>Value of Y outside Function ".$y; //Error
showData(); //Function Called
?>
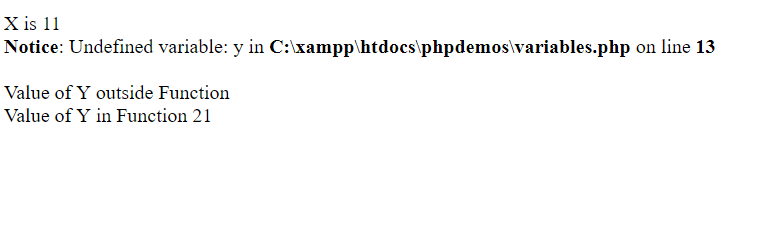
And if we use variable x inside the function, that will also result in an error.
<?php
$x=11;
echo "<br>X is ".$x; //. is for concatenate
function showData()
{
$y=21;
echo "<br/>Value of Y in Function ".$y;
echo "<br/>Value of X in Function ".$x; //Error
}
echo "<br/>Value of Y outside Function ".$y; //Error
showData(); //Function Called
?>
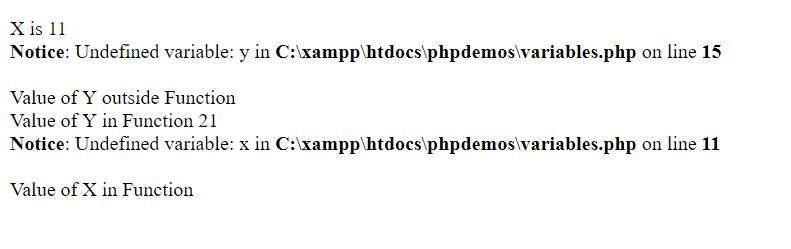
But if you want to use the variable x declared outside the function you have to use global keyword in function body.
<?php
$x=11;
echo "<br>X is ".$x; //. is for concatenate
function showData()
{
global $x;//Now you can use x
$y=21;
echo "<br/>Value of Y in Function ".$y;
echo "<br/>Value of X in Function ".$x; //Error
}
//echo "<br/>Value of Y outside Function ".$y; //Error
showData(); //Function Called
?>
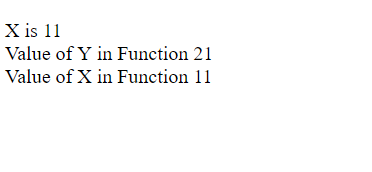
Another storage class for variable is static variables. static variables remains their value even after execution of function. (Like C Language)
<?php
function staticDemo()
{
$x=20;
static $y=20;
echo "<br/> X ".$x. " and Y ".$y;
$x++;
$y++;
}
staticDemo();
staticDemo();
staticDemo();
?>
In above example $x is non-static and $y is static. We have called staticDemo() function 3 times. Each time $x is initialized with 20 but static variable $y is initialized only once. value of $y (static) continuously increase in each call.
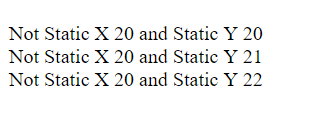
When constant values are required in php you can define the with define().
<?php
define('LANGUAGE','PHP');
define ('PI', 3.14);
echo "Value of PI is (Constant) ".PI;
echo "<br/>Langauge is ".LANGUAGE;
define('PI',33); //ERROR
$PI=3; //its ok, its variable not constant
echo $PI; //value of variable
?>
Constant values in PHP
