Using Layouts in Swing JFrame
As Swing is used for GUI in Java. We can develop a GUI Based Application using Swing. Hereby we have sampled a program for JFrame. We have also applied BorderLayout and also setBounds for designing the form. In our class we have three JPanels. JFrame is set to BorderLayout and top, bottom and center panels are added. In Top Panel we have applied FlowLayout with Center Alignment and added the JLabel for title of the Form. In Center Panel we have applied setLayout(null) and set the controls using setBounds at absolute position.
package jdbcdemo;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JSpinner;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class InsertData extends JFrame {
JLabel lblTitle;
JLabel lblWebsiteName;
JTextField txtWebsiteName;
JLabel lblWebsiteDetails;
JTextArea txtWebsiteDetails;
JLabel lblWebsiteRank;
JSpinner spnWebsiteRank;
JButton btnSubmit;
JPanel pnlTop;
JPanel pnlCenter;
JPanel pnlBottom;
public InsertData(){
setLayout(new BorderLayout());
pnlTop= new JPanel();
pnlCenter= new JPanel();
pnlBottom= new JPanel();
this.add(pnlTop,BorderLayout.NORTH);
this.add(pnlBottom,BorderLayout.SOUTH);
this.add(pnlCenter,BorderLayout.CENTER);
pnlTop.setLayout(new FlowLayout(FlowLayout.CENTER));
lblTitle= new JLabel("WEBSITE MANAGEMENT");
lblTitle.setFont(new Font("Serif", Font.PLAIN, 24));
pnlTop.add(lblTitle);
pnlCenter.setLayout(null);
lblWebsiteName= new JLabel("Enter Webiste Name");
lblWebsiteName.setBounds(10,10,150,30);
pnlCenter.add(lblWebsiteName);
txtWebsiteName= new JTextField();
txtWebsiteName.setBounds(180,10,290,30);
pnlCenter.add(txtWebsiteName);
lblWebsiteDetails= new JLabel("Enter Webiste Details");
lblWebsiteDetails.setBounds(10,60,150,30);
pnlCenter.add(lblWebsiteDetails);
txtWebsiteDetails= new JTextArea();
txtWebsiteDetails.setBounds(180,60,290,130);
pnlCenter.add(txtWebsiteDetails);
lblWebsiteRank= new JLabel("Select Webiste Rank");
lblWebsiteRank.setBounds(10,220,150,30);
pnlCenter.add(lblWebsiteRank);
spnWebsiteRank= new JSpinner();
spnWebsiteRank.setBounds(180,220,90,30);
pnlCenter.add(spnWebsiteRank);
btnSubmit= new JButton("Save");
btnSubmit.setBounds(180,260,90,50);
pnlCenter.add(btnSubmit);
setSize(700,600);
setDefaultCloseOperation(DISPOSE_ON_CLOSE);
btnSubmit.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
// TODO Auto-generated method stub
String webname= txtWebsiteName.getText().trim();
String webdetails= txtWebsiteDetails.getText().trim();
String webRank= spnWebsiteRank.getValue().toString();
//Here you can process your values
System.out.println(webRank);
}
});
}
public static void main(String []a) {
InsertData mainform= new InsertData();
mainform.setVisible(true);
}
}
In setBounds(x,y,w,h) , x is x (column) position of the control, second parameter y (row) position of the control , w is width of control and h is height of the control. If you do not provide setBounds in case of setLayout(null) your Control will not display in the Frame.
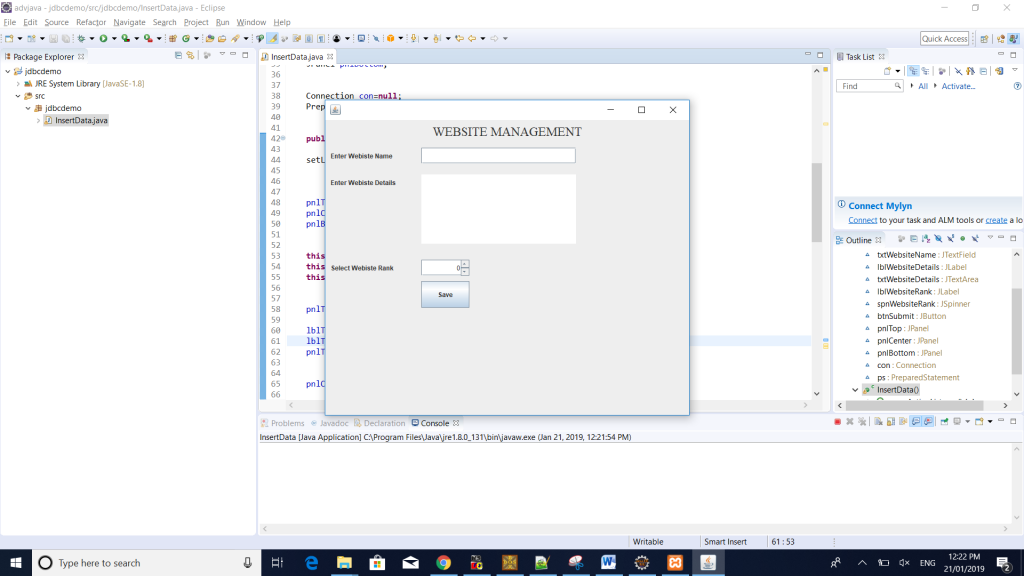