Switch Case in C Language
As an alternate of if..else in some cases we can use switch.case. Switch case is also a decision making control. We can use Switch.. case with Integral Data Type (int/char/short etc..) only, Switch case not be used with float/double or string data type
switch(variable)
{
case val1 :
Statements for val1..
break;
case val2 :
Statements for val2;
break;
default:
works like else (executes when none of above case is executed)
}
default is optional, default part executes when none of mentioned cases are true. Break is also optional but we use don’t write break in any case, next case will also execute. Means once execution enters in any case it stops when first break is founded.
#include<stdio.h>
#include<conio.h>
void main()
{
int day;
clrscr();
printf("\nEnter Day No :");
scanf("%d",&day);
switch(day)
{
case 1:
printf("\nSunday");
printf("\nHoliday");
break;
case 2:
printf("\nMonday");
break;
case 3:
printf("\nTuesday");
break;
default:
printf("\nInvalid Day");
}
getch();
}
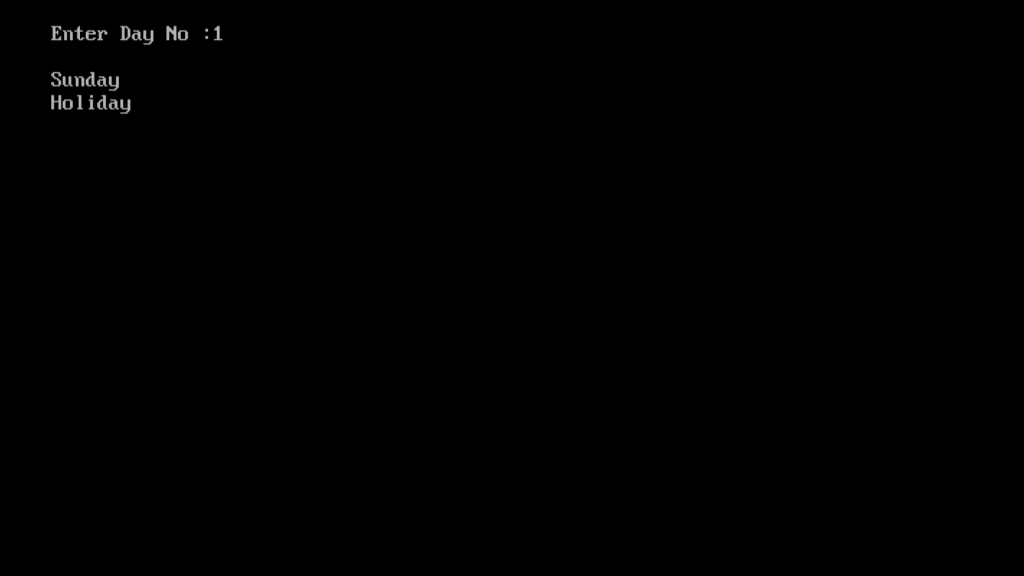
#include<stdio.h>
#include<conio.h>
void main()
{
int no;
clrscr();
printf("\nEnter No :");
scanf("%d",&no);
switch(no)
{
case 1:
printf("One ");
//We have not written break here so case 2 will also execute
case 2:
printf("\nTwo");
break;
case 3:
printf("\nThree");
break;
default:
printf("\nInvalid No");
}
getch();
}
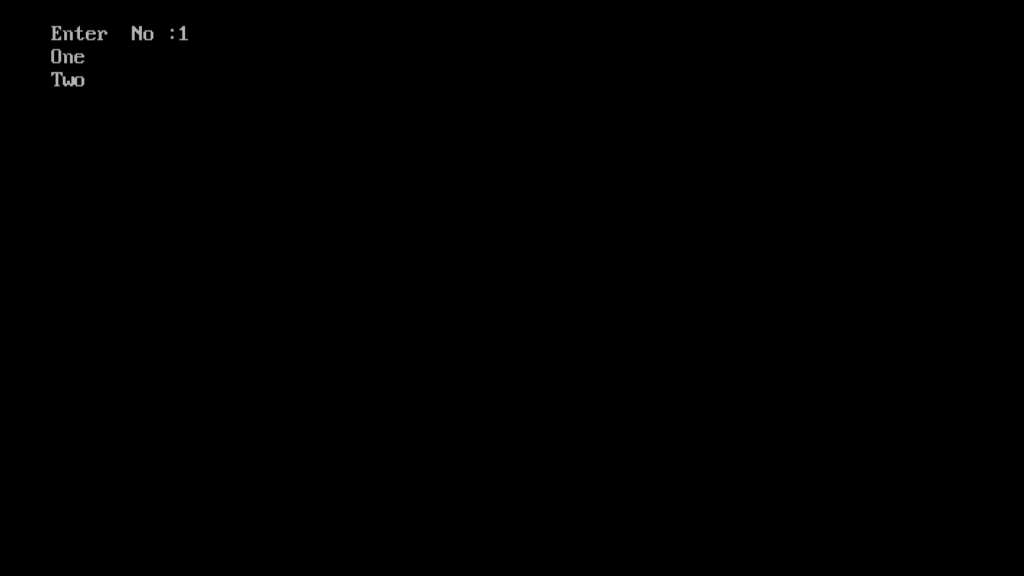
We can replace switch case with if like
if(day==1)
{
}
else if(day==2)
{
}
else if(day==3)
{
}
else
{
}