Pointer with Example in C language
pointers are special type of variables in C Language that stores the Address of another variable:
int *p=&a; //Here address of a will be stored in P
We can access the value of stored variable using * (ex *p). Now a days they are mostly used for Programming in Micro Controllers. We can use %u type specifier (unsigned) to print address block.
#include<stdio.h>
#include<conio.h>
void main()
{
int *p;
int x=20,y;
clrscr();
p=&x ; // Address of X will be stored to P;
printf("\nAddress of X is %u and Value is %d",p,*p);
//*p will print the value of x
y=30;
p=&y;
//Now P points to Y
printf("\nAddress of Y is %u and Value is %d",p,*p);
getch();
}
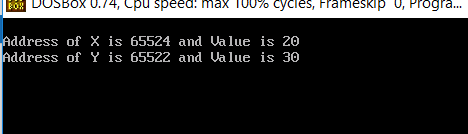
Pointer of any data type always uses 2 Bytes because it is always Integer.
#include<stdio.h>
#include<conio.h>
void main()
{
float x=9.4;
float *p=&x;
clrscr();
printf("\nSize of X is %d Bytes ",sizeof(x));
printf("\nSize of P is %d Bytes ",sizeof(p));
getch();
}
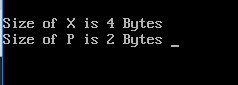