if statement in C Language
For Decision making we can use if statement in C Language. If statement is used for conditional execution of statements. Basic Syntax of is:
if(condition)
{
true-part here
}
if(condition)
{
true-part;
}
else
{
false-part;
}
if(condition 1)
{
True-Part of Condition 1
}
else if(condition 2)
{
True Part of Condition 2
}
else
{
false-part
}
#include<stdio.h>
#include<conio.h>
void main()
{
int x;
clrscr();
printf("\nEnter No :");
scanf("%d",&x);
if(x>0)
{
printf("\nX is Greater Than Zero");
}
getch();
}
/*
if we provide positive value of x, then output will be X is Greater Than Zero, otherwise nothing in output.
For x=0 it will not display anything as no condition is fulfilled.
*/
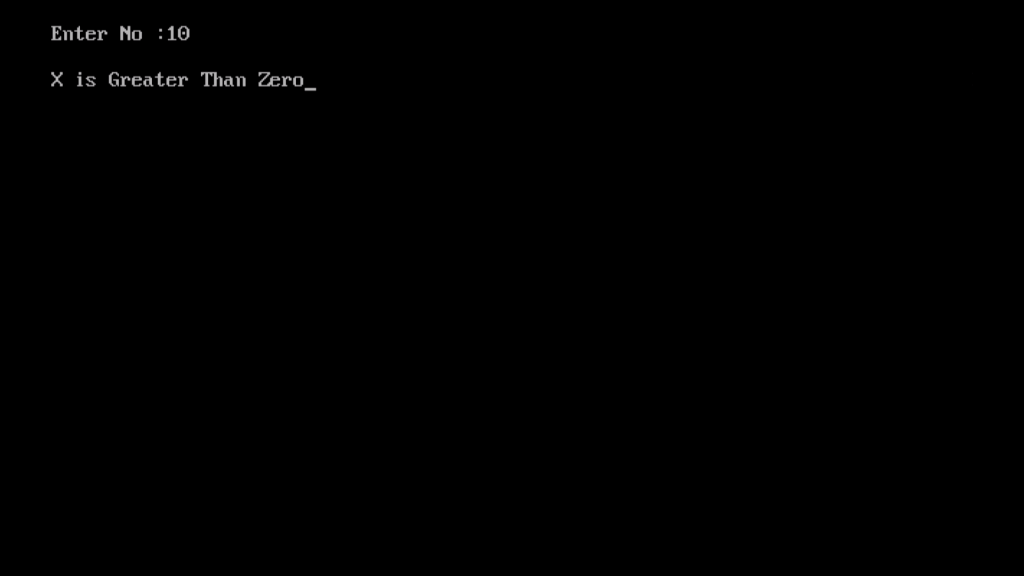
#include<stdio.h>
#include<conio.h>
void main()
{
int x;
clrscr();
printf("\nEnter No :");
scanf("%d",&x);
if(x>0)
{
printf("\nX is Greater Than Zero");
}
else
{
printf("\nX is Neg.");
}
getch();
}
/*
For this program if we provide 0 or negative values, then output will be X is Neg". And for Positive value it will show X is Greater than Zero
*/
Now we will discuss about multiple conditions in If. It is also called else if ladder.
#include<stdio.h>
#include<conio.h>
void main()
{
int x;
clrscr();
printf("\nEnter No :");
scanf("%d",&x);
if(x>0)
{
printf("\nX is Greater Than Zero");
}
else if(x<0)
{
printf("\nX is Neg.");
}
else
{
printf("\nX is Zero");
}
getch();
}
/*
Here if x is positive then Output :X is Greater Than Zero
if x is Negative then output : X is Neg
and if we provide x 0 then output will be X is Zero
*/
In above example if we do not provide else part and enter 0 to value of x then Output will be nothing, as none of two conditions are fulfilled.
We will discuss many interesting topics related to conditions. Do not forget to subscribe to our blog to get updated posts.