Fibonacci Series in C Language
Fibonacci Series a special Numerical Series where Number is addition of previous two numbers. Fibonacci Series starts from 0 and 1.
0 1 1 2 3 5 8 13 21 …..
Hereby we have demonstrated the Fibonacci Series Program in C Language using For Loop. Any type of Iteration can be used for the same. You can also use While Loop or Do While or goto for the same purpose.
#include<stdio.h>
#include<conio.h>
void main()
{
int i,n;
int f=0,l=1,sl;//f final step, l last step, sl second last step
clrscr();
printf("\nHow Many Steps of Fibb. Series You Want ?");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
printf("%4d",f);
sl=l; //last will be second last now
l=f; //final will be last step
f=l+sl; // new final is addition of last and second last
}
getch();
}
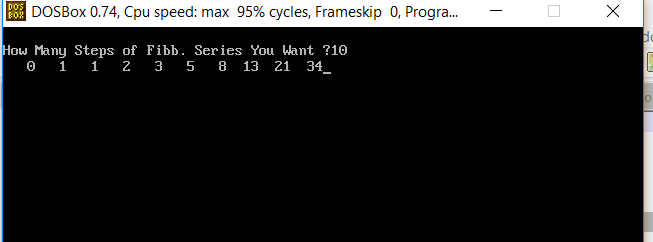
In above Program we have counted the steps of Fibonacci Series. We can modify the same program for the limit of Number value like :
#include<stdio.h>
#include<conio.h>
void main()
{
int i,n;
int f=0,l=1,sl;//f final step, l last step, sl second last step
clrscr();
printf("\nEnter Value Upto Fibonacci Series to Print ?");
scanf("%d",&n);
for(i=1;f<=n;i++)
{
printf("%4d",f);
sl=l; //last will be second last now
l=f; //final will be last step
f=l+sl; // new final is addition of last and second last
}
getch();
}
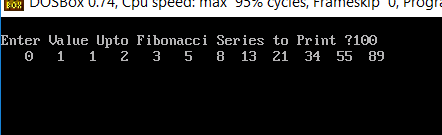