Basics of Exception Handling in Java
Exceptions are the cases, where error may occur on conditional basis at run time. ex. Divide by Zero if use provide inputs that lead to Division by zero. If any error occurs at run time then JRE throws exception and stops the execution of the program from same line. But if you manage exceptions using try and catch, further execution will continue. Whenever error occurs, execution goes to catch block. And statements below catch block are executed normally.
class ExceptionDemo1{
public static void main(String []a){
int x,y,z=0;
try{
x=Integer.parseInt(a[0]);
y=Integer.parseInt(a[1]);
z=x/y;
System.out.println("Ans :"+ z);
}catch(Exception e){
e.printStackTrace();
}
System.out.println("Will Always Execute");
}
}
Various Cases of Execution
When we provide no arguments or single argument at run time there will be exception like ArrayIndexOutOfBoundsException, as we have used a[0] and a[1].
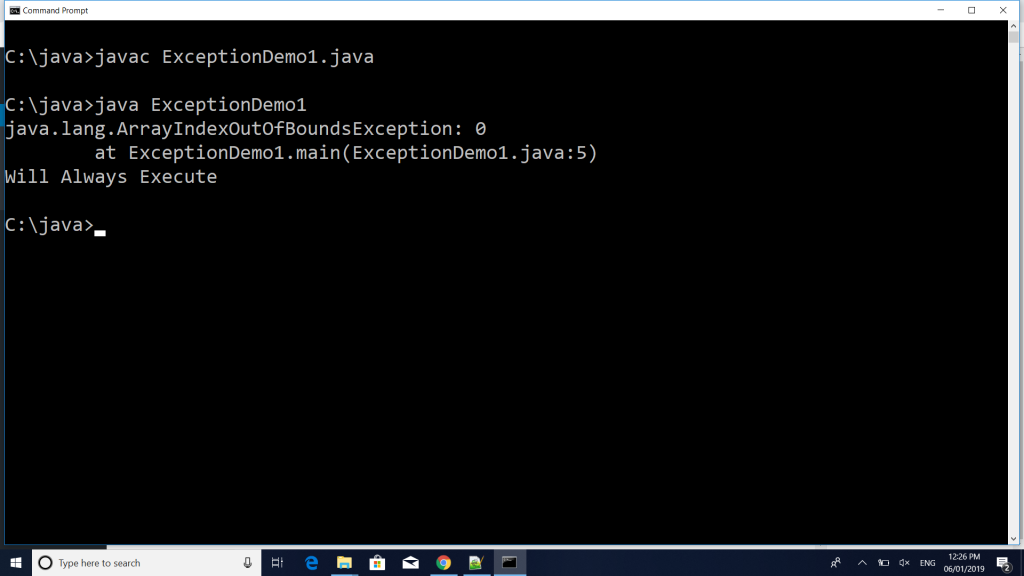
When we provide two values but Second Argument is 0, it will throw ArithmeticException for Divide By Zero.
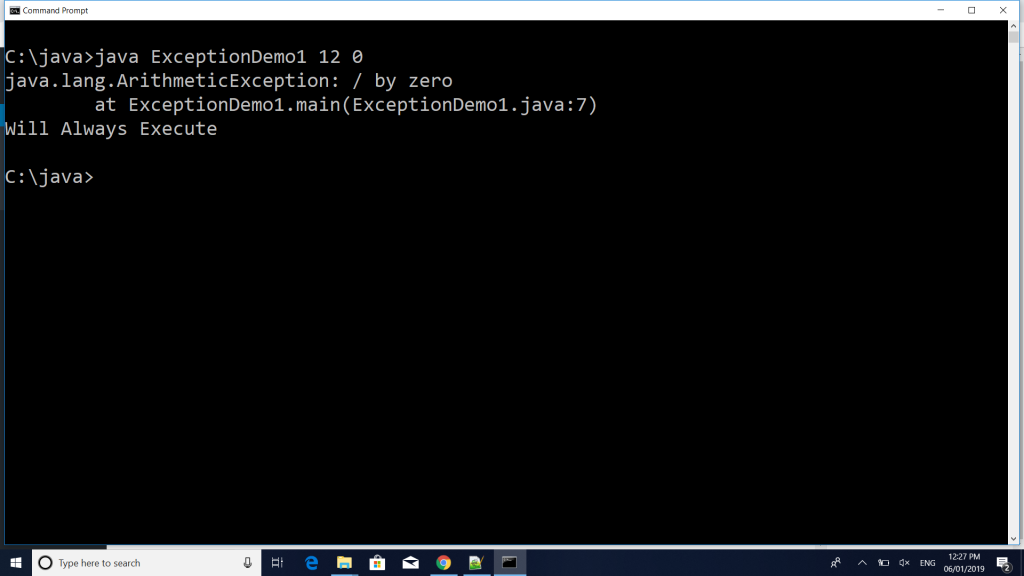
If we provide all two arguments proper, then it will not throw any exception.
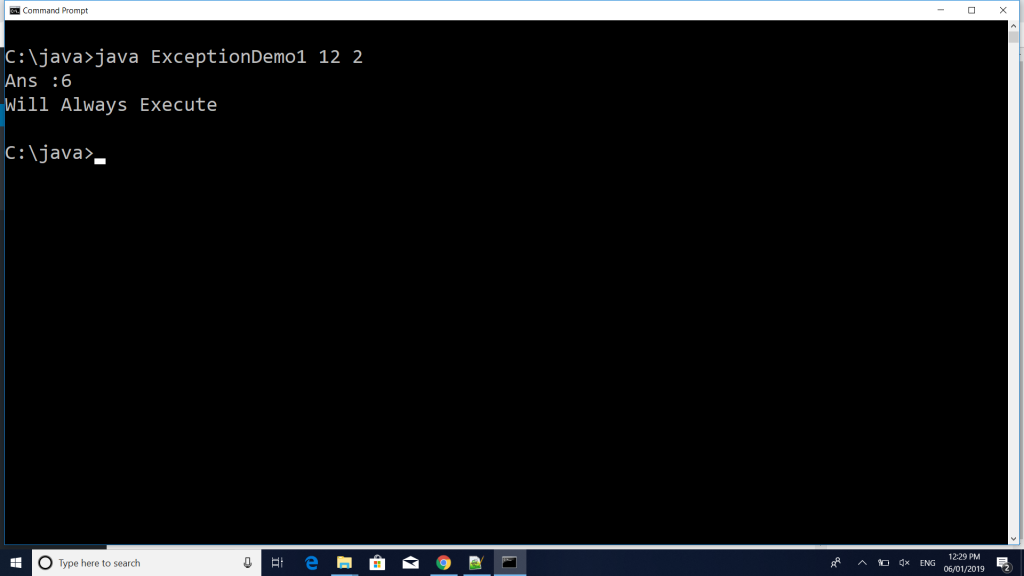
In above three cases you can note that ‘Will Always Execute’ is shown every time due to try.. catch block. But if you remove try..catch block and provide invalid values like 0 as second parameter, ‘Will Always Execute’ will also not shown.
class ExceptionDemo2{
public static void main(String []a){
int x,y,z=0;
x=Integer.parseInt(a[0]);
y=Integer.parseInt(a[1]);
z=x/y;
System.out.println("Ans :"+ z);
System.out.println("Will Always Execute");
}
}
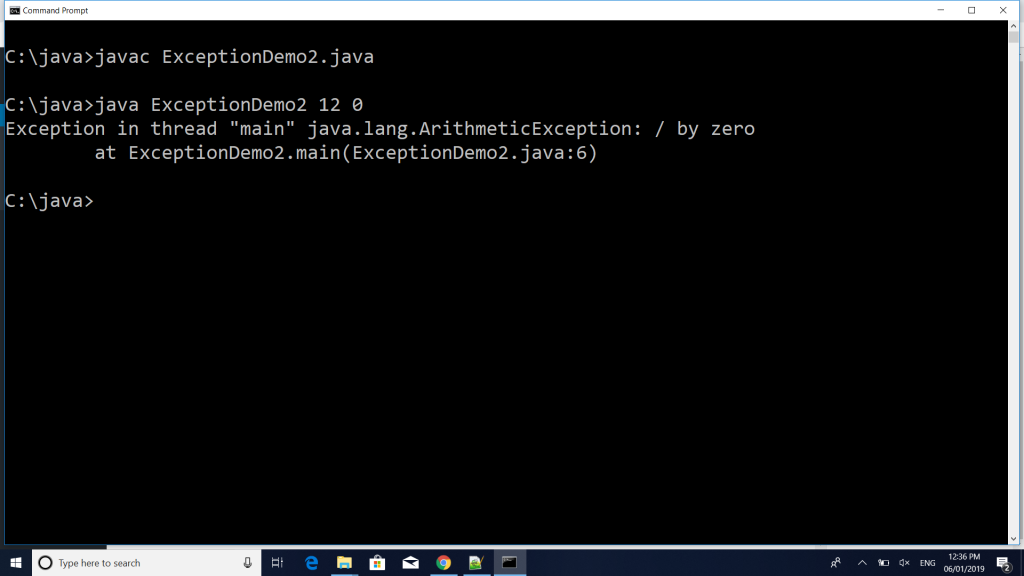
Don’t forget to subscribe to get more details in future.